What is JavaScript?
JavaScript is a lightweight programming language that allows a developer to create dynamic web pages. All those animated graphics, video jukeboxes, interactive maps you see on websites, those were most probably created using JavaScript. JavaScript is a scripting language that supports object-oriented and procedural programming styles.
It runs on the client-side, which allows developers to determine how a web page will react to certain events.
The History of JavaScript
JavaScript was created by Brendon Eich in 1995. Eich was employed by Netscape to create a scripting language that not only allowed web pages to be more dynamic, but was also easy for non-developers (designers) to pick up and learn.
Netscape decided that their new scripting language, had to have a syntax similar to Java, as Java was gaining popularity at the time. Eich wrote the first JavaScript prototype in only 10 days! JavaScript's first name was Mocha, which was later changed to LiveScript, due to marketing and trademark reasons. JavaScript was first integrated in Netscape Navigator 2.0, in November 1995. LiveScript was later changed to JavaScript in December 1995.
Evolution of JavaScript
After the release of JavaScript, Microsoft implemented the same language under a different name, JScript. Netscape decided to standardise JavaScript. The ECMA was asked to host the standard. The ECMA is an industry assocation founded in 1961, that deals with the standardisation of information and communication systems.
Work on the standard started in November 1996, this specification was called ECMA-262. Due to trademark reasons, the ECMA committee could not use the name JavaScript. The official name therefore became ECMAScript. JavaScript is the commercial name.
The ECMA-262 specification defined a standard version for JavaScript:
- JavaScript is a lightweight, interpreted programming language.
- Designed for creating network-centric applications.
- Complementary to and integrated with Java.
- Complementary to and integrated with HTML.
- Open and cross-platform
Now that you know what JavaScript is and it's history, let's get to coding.
Hello World JavaScript App
Your first JavaScript app is going to be a simple app that will display Hello World on a Web browser.
JavaScript can be applied to a HTML web page in two ways:
- It can be embedded within HTML code.
- It can be external and referenced by a HTML web page.
We are going to look at both of these approaches in our Hello World App.
The Embedded Approach:
JavaScript can be embedded with HTML, through the use of the <script> tag. We are now going to create our first embedded JavaScript app.
Open notepad or any text editor of your choice.
The following code displays an alert box with the message 'Hello world', in your browser when you click the Click Me button.
In the code above, our JavaScript code in found within the <script>....</script> tag. The script tag alerts the browser program to start interpreting all the text between these tags as a script.
Between the script tags there we have a function called displayHello(). In JavaScript functions are created by using the keyword function followed by the name of the function. Our displayHello function is responsible for displaying an alert box with the message 'Hello World'. In order to call this function we have to make use of events. In our example we make use of the onclick event, which triggers our function when a button is clicked. Refer to line 17, we have a button which triggers our function when it is clicked. In order to use the onclick event we use the keyword onlick followed by the JavaScript function we would like to call when the button is clicked.For a list of other JavaScript events visit the following site https://www.w3schools.com/js/js_events.asp.
To test our Hello World app. Open the HTML file and it should open in your browser. You should see the following:
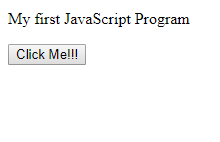
When you click the button the following message should appear:
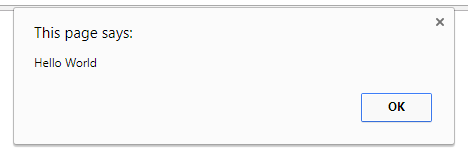
The External Approach
Now we will look at the external approach to JavaScript. We will use the same Hello World example as the above and we will tweak it to follow to the external approach.
As you can see in the code above the script tags are a little different. We have a src element which points to our JavaScript file's location, which contains our JavaScript code. Our button tag also contains an event onclick which will call the displayHello() function which is defined in our external code.
In order to create an external JavaScript file we will need to do the following:
- Open up a text editor, like Notepad.
- Write you JavaScript code.
Note: it is good practice to save all your JavaScript code in a folder such as js, it makes code maintenance a lot easier.
Copy the following code and save your while as yourfilename.js. Remember to reference your file within your html.
As you can see this is the exact same JavaScript code as before. The only difference is that the code is contained within it's own file which makes code maintenance a breeze when your JavaScript becomes long and complicated.
We have a function called displayHello() which, when called will display an alert box with the message "Hello World".
Calculator App
We are now going to create something a little more exciting than an alert box with a message. We are going to create our very own simple calculator using JavaScript! Our calculator will take in two numbers, the user will then choose the operation to perform on the two numbers and then the result will be displayed to the user.
Let us begin.
For our calculator app we will be making use of the external JavaScript approach, since our code is going to be a little more complicated than the Hello World app.
Let's break it down
The code above is a dropdown box which contains the mathematical operations the user can choose from.
<input type = "text" id = "num2"/> this is where the user will enter the second number.<button onclick = "calculate()">Calculate Me!</button> the onclick event is triggered when the button is clicked, calling the calculate function into action.
Here we have one function called calculate which will be called into action when called by our "Calculate Me!" button is clicked. JavaScript is loosely typed, which means the data type of variables are not needed when creating variables. Variables are is simply a storage location. The data type is determined by the value it holds.
We have two variables num1 and num2 which is identified by the keyword var, these two variables hold the two numbers the user has entered. We retrieve the entered number by using the JavaScript function document.getElementById().value. This function gets the value of an element with the specified ID passed as an argument. In our example we pass num1 and num2 as our IDs, num1 is the id of our first text box which we specify by using id = "num1" in our input tag and "num2" is the id of our second text box.
In order to perform a calculation we need to have numbers. When we get values from text boxes more often than not these values are strings. The variables var val1 = parseInt(num1); and var val2 = parseInt(num2);will contain our inputs in the form of numbers. To convert strings to numbers we use the parseInt() function which takes the string value as an argument and then converts our string value to a number.
We then have a variable called operation, var operation = document.getElementById("operation").value; which contains the selected operation. Again we use the document.getElementId().value function to retrieve the selected operation from our dropdown box.
We are now ready to divulge into the actual calculations of our numbers.
In order to determine what operation to perform on user's numbers, we make use of a conditional statement, the if statement. We use the if statement to specify a block of code when a certain condition is true. In our example we have a number of if statements that will execute a block of code only when operation variable equals a certain value for example we have
The code within the if statement will only be executed when operation equals add (operation variable contains value add). We a number of if statements used to determine which operation the user has selected, which in turn determines which operation to perform on the numbers.
In each if statement we have the following
As you can see we now make use of a if....else statement. The else in our statement specifies the block of code to executed when a certain condition is false. Now let us break down our example.
This block of code is used to determine whether the number entered by the user are indeed valid numbers. The function isNaN() (is Not a Number) checks to see whether or a value is a number of not. If the value is not a number it will return true, if the value is a number it will return true. Therefore in our example we are saying if num1 is not a number or if num2 is not a number then display our alert box. || stands for OR, which means one or all conditions can be true in order for the code block within the statement to be executed.
Our else block, which executes only when our condition in the if statement is false has a variable called results, which will store the result of our operation, in this case the addition operation.
The line document.getElementById("result").innerHTML = "Result: "+result will display our result in the HTML element with the id result (<p id ="result"></p>).
document.getElementById().innerHTML displays the resulting string within the HTML element of the specified ID.
As you can see in our code we have similar four if statements for the four mathematical operations, we already when the if statement operation, so you should be able to break down and understand the other 3. We will however look at the following block of code:
As you can see this is similar to the add if statement that we already dissected. We are going to focus on the following however:
In mathematics, we when we divide a number by zero, it is undefined. So we need to check if our second number if 0 and if it is we need to then display a message to the user.
This checks if the second number the user provided as input is 0. If it is we set our result variable to "Tis impossible".
Our else statement is executed when the second number is not 0. We are then able to divide our two numbers and the result of the operation is then stored in our result variable.
Our result is then displayed to the user in the following statement document.getElementById("result").innerHTML = "Result: "+result;
Now it's time for to test and play with your calculator.
When you open your calculator in your browser you should see the following:

Enter values, select an operation then click Calculate me:

When you enter an invalid number
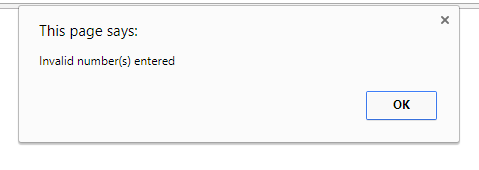
Present state of JavaScript
JavaScript is currently being utilised in many websites and we applications. Your mobile applications could secretly be created with JavaScript. A number of JavaScript frameworks have been created to improve JavaScript have revolutionised web development, even allowing for the development of hybrid mobile and desktop applications. This means all you need to do write one set of code for your application an it can run multiple devices on multiple operating systems.
Some of these libraries include:
- Angular
- Ionic
- Node.js
- Node.js
- Backbone.js
- Knockout.js
At ZonkeTech we make use of most of these libraries due to their simplicity. They make web development as well as mobile development a breeze.
It's quite evident that JavaScript is here to stay and will eventually become your one stop general purpose programming language. This can be seen by the introduction of powerful JavaScript libraries, which make single page web apps and hybrid app development a breeze. Data from databases can be transported and stored easily through the use of JSON (JavaScript Object Notation). JavaScript has become and is still evolving not only into a front-end, web language but it's also evolving into a language for the interaction between a large number of modern devices.
JavaScript has become an interesting target for other languages, when it comes to compilation. For instance, video games, usually written in C++, can now be ported to the browser with a lot more ease than before. This is due to the now powerful JIT JavaScript VM.
Now go forth, and build cool stuff.