What is C#?
C# is an objected-oriented language created by Microsoft. It enables developers to build robust applications that run on the .Net Framework. C# can be used create Windows client applications, XML Web services, distributed components, client-server applications, database applications, among others.
It's easy to learn and anyone who is familiar with Java, C or C++ will be able to grasp in no time.
C# programs run on the .Net Framework. The framework includes a virtual execution system called the common language runtime (CLR). Source code written in C# is compiled into an intermediate language (IL) that conforms to the CLI specification.
When the C# program is executed, the assembly is loaded into the CLR, which might take various actions based on the information in the manifest.
The History of C#
C# was created by Anders Hjelsburg. In January 1999, Hjelsburg formed a team to create a language to overcome the flaws of many of the programming languages of the time. C# was originally called Cool (C-like Object_Oriented Languages) and was later renamed C#. The name C# was inspired by the musical notation where a sharp (#) indicates that a written note must be semitone or high pitched. C# was publicly announced in July 2000. It was later officially released in 2002. The latest version is 7.0.
Develop your first C# app
What you will need:
A PC with Visual Studio installed.
The latest .Net Framework installed.
Developing a Hello World Console App:
Open Visual Studio.
In the top menu bar click File → New → Project
Next click Visual C# on the left side bar, select Console App (.Net Framework)
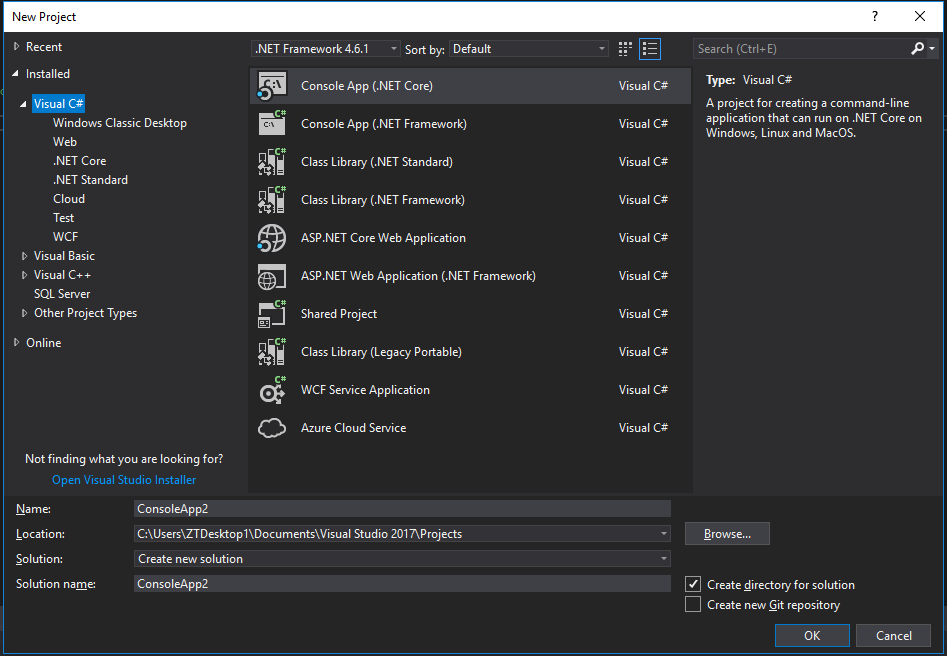
Name your project and then click OK.
Visual Studio will create everything you need for you, including a file called Program.cs.
You will see the following in your Program.cs file
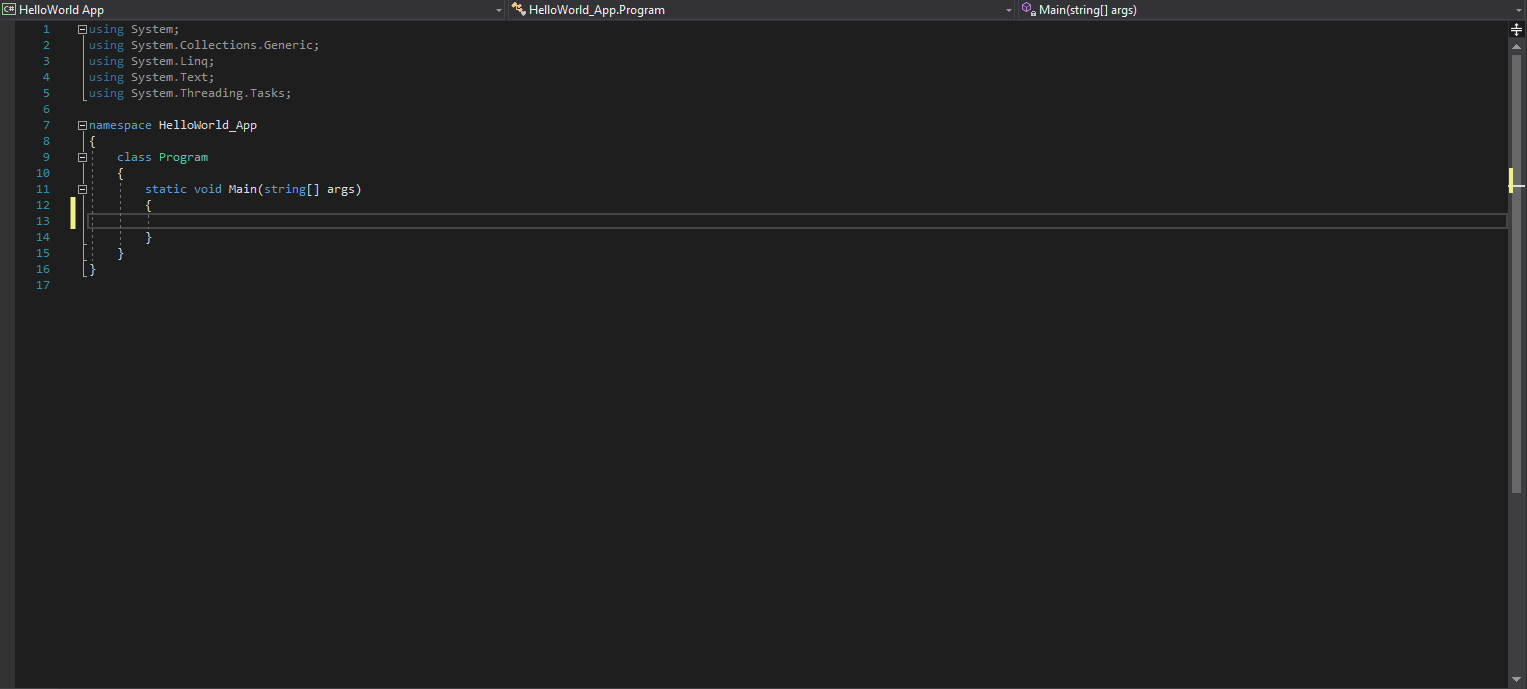
Now it's time to code!
If you run the application, by pressing F5, you should see the following:
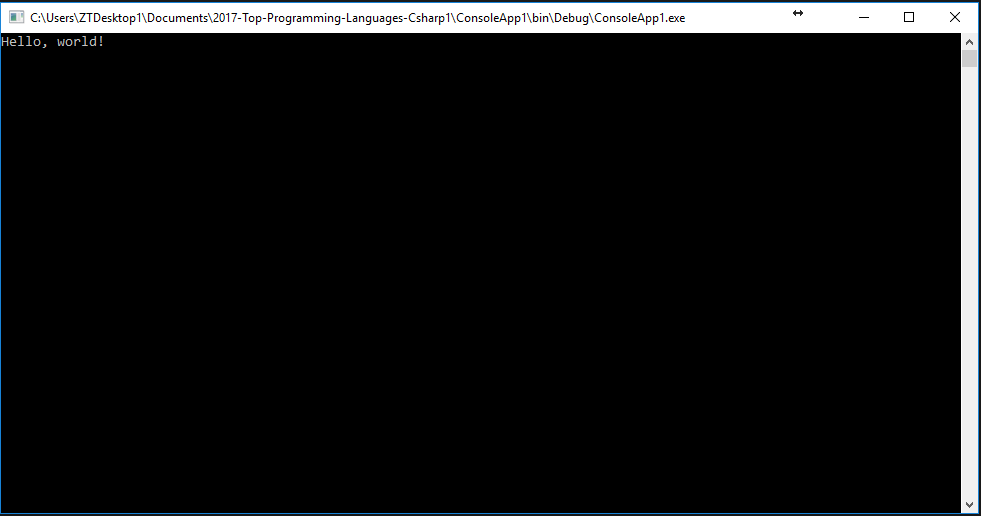
Let's break it down:
A C# program consists of the following:
- Methods
- A main method
- Statements and Expressions
- Comments
The first line in our program using System imports the namespace System. A namespace is a collection of classes. Therefore we are importing all the classes in the System namespace, so that we can use them in our app.
The next line namespace ConsoleApp1 is the namespace declaration. The name of our namespace is ConsoleApp1 and it includes our class Program.
The next line, is the class declaration. The name of our class is Program. It contains the data and method definitions that your program uses. Methods define the behavior of the class.
The next line defines the Main method. This is the application's entry point. This is the first method that is excuted when your application first runs. In order for your application to run you need to have a Main method. The Main method states what the class does when executed.
The next line Console.WriteLine("Hello, world!"), prints hello world in the console. Console is the class we import from the System namespace. WriteLine is the method we call from the Console class which prints Hello World on the console.
The last line Console.ReadLine() . This makes the program wait for a key press and it prevents the screen from running and closing quickly when the program is launched.
Congratulations you have just created your first C# app.
Developing a console calculator app
Open Visual Studio.
Click File → New → Project
In the Project dialog window select Visual C#, like before.
Like before select Console App (.Net Framework) and name your project
Click OK.
Modify your Program class:
Now let's break it down:
The line Program prog = new Program() creates a new object for our application. We need to create an object for our class in order to call and execute non-static methods from within our Main static method. Remember that the Main method executes the behaviour of our class.
The next line int num1, num2, operation are our variables that we are going to use to hold user input. A variable is a storage location in memory.
The next line Console.WriteLine("Press any key to continue") tells the user to press any key, in order to continue the execution of the application.
The next line while (!(Console.ReadKey(true).Key == ConsoleKey.Escape)) is the start of the while. It will loop and execute the code in it until the condition is false. In our case it will loop until the user presses Esc. When Esc is pressed the application will close. Console.ReadKey(true).Key reads the key pressed by the user.
The next two lines Console.WriteLine("Press ESC to stop") and Console.WriteLine("Enter first number") prompts the user on what to do.
The next line num1 = int.Parse(Console.ReadLine()) reads the user input and stores it in the variable num1. int.Parse() converts the the user input Console.ReadLine() input an integer.
The next couple of lines tells the user how to choose an operator for their calculation
The next line operation = int.Parse(Console.ReadLine()) reads and stores the user input in the variable operation.
The next set of statements are known as if... else if. We use this to determine which operation the user selected.
The if statement check to see if the operation method is equal to 1, if it is it proceeds to call the add method. As you can see we had to create an object for our calculator class for that our static main method can call our non static add method. Also we pass to values to the add method, these are the numbers the user enter previously. If operation is not 1 then it proceeds to the next else if. It checks to see if operation is equal 2 to if it is, it will exceute that code block, if not it moves on to the next else if. If the code has moved through all the else ifs and reaches the else block, it will then exceute what is in it, as all conditions before that was false. In our case the user will then be notified of their invalid choice.
We then have our operation methods
The add method takes in two numbers, adds the two numbers and then displays the result to the user.
The subtract method takes in two numbers, subtracts them and then displays the result to the user.
The divide method takes in two numbers, divides them and then displays the result to the user. If the second number is zero a message is displayed to the user indicating to the user that dividing a number by zero is not recommended.
The multiply method takes in two number, multiplies them and then displays the result to the user.
When you run your calculator you will see the following:
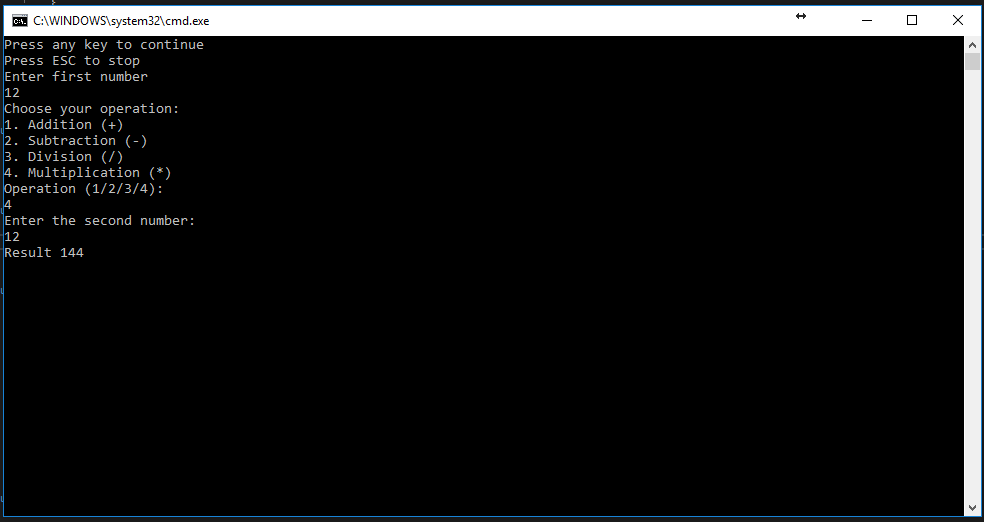
The state of C#
C# is one of the most popular languages currently. I has evolved quite a bit since its release in 2002. It has become a flagship language in web and mobile development and now that MIcrosoft has shifted its focus to artificial intelligence, it is quite possible that it will become a key feature in AI. C# is here to stay and it will only become more and more powerful.